Building a Simple BMI Calculator GUI with Go and Fyne
Introduction to Go Programming: Building a Simple BMI Calculator GUI
Go Programming (also known as Golang) is a powerful and efficient programming language that has gained popularity for its simplicity and performance. While Go is often used for backend and systems programming, it is also capable of creating desktop GUI applications. In this Go Programming tutorial, we will guide you through setting up Go, creating a simple BMI (Body Mass Index) calculator using the Fyne GUI library, and understanding the basics of GUI development in Go. This Go programming tutorial is perfect for beginners with no prior experience in Go or GUI development.
What We Will Build
We will build a simple BMI calculator that allows users to input their weight in kilograms and height in meters. The application will then calculate the BMI and provide a health category (e.g., Underweight, Normal weight, etc.) along with a helpful tip based on the BMI result. Additionally, the application will feature a light/dark theme toggle for user preference.
Prerequisites
Before we start, make sure you have the following installed on your machine:
- Go: If you haven’t installed Go yet, you can download it from the official Go website. Follow the instructions for your operating system.
- Fyne: Fyne is a cross-platform GUI library for Go that makes it easy to create beautiful and responsive desktop applications. We will install it as part of this tutorial.
Step 1: Setting Up Your Go Environment
- Install Go:
- Download the Go installer from the official site.
- Run the installer and follow the prompts.
- Once installed, you can verify the installation by opening a terminal and typing:
go version
- You should see something like
go version go1.16.3
(or whichever version you installed).
- Set Up Your Workspace:
- Go uses a workspace structure where all your projects reside. By default, your workspace should be located in
~/go
(on Unix systems) orC:\Users\YourName\go
(on Windows). - Create a directory for your new project:
mkdir -p ~/go/src/github.com/yourusername/bmi-calculator cd ~/go/src/github.com/yourusername/bmi-calculator
Step 2: Installing the Fyne Library
Now that your Go environment is set up, let’s install the Fyne library.
- Install Fyne:
- In your project directory, run the following command to install Fyne:
go get fyne.io/fyne/v2
- This will download and install Fyne and its dependencies.
- Create Your Main Go File:
- In your project directory, create a new file named
main.go
:touch main.go
- Open
main.go
in your preferred text editor or IDE.
Step 3: Writing the BMI Calculator Code
Let’s dive into writing the code for our BMI calculator. The code is designed to be simple and easy to understand, even for those new to Go.
The Code
package main
import (
"fmt"
"fyne.io/fyne/v2"
"fyne.io/fyne/v2/app"
"fyne.io/fyne/v2/container"
"fyne.io/fyne/v2/theme"
"fyne.io/fyne/v2/widget"
"strconv"
)
func calculateBMI(weightKg, heightM float64) (float64, string, string) {
bmi := weightKg / (heightM * heightM)
fmt.Println(bmi)
var category, tip string
switch {
case bmi < 18.5:
category = "Underweight"
tip = "Consider increasing your calorie intake with nutrient-rich foods
and consult with a healthcare provider for a plan to reach a healthy weight."
case bmi >= 18.5 && bmi <= 24.9:
category = "Normal weight"
tip = "Maintain your healthy lifestyle with a balanced diet and regular physical activity."
case bmi >= 25 && bmi <= 29.9:
category = "Overweight"
tip = "Incorporate more physical activity into your
routine and watch your portion sizes to manage your weight effectively."
case bmi >= 30 && bmi <= 34.9:
category = "Obesity (Class 1)"
tip = "Focus on a healthier diet and regular exercise.
It’s advisable to consult with a healthcare provider for personalized advice."
case bmi >= 35 && bmi <= 39.9:
category = "Obesity (Class 2)"
tip = "Medical advice is recommended to develop a weight loss plan,
as well as to monitor any related health conditions."
default:
category = "Severe Obesity (Class 3)"
tip = "Seek professional medical assistance to address potential
health risks and to develop a comprehensive weight management plan."
}
return bmi, category, tip
}
func main() {
app := app.New()
w := app.NewWindow("BMI Buddy")
w.SetFixedSize(true)
w.Resize(fyne.NewSize(700, 500))
weight := widget.NewEntry()
height := widget.NewEntry()
bmiText := widget.NewLabel("")
categoryText := widget.NewLabel("")
tipText := widget.NewLabel("")
themeStyle := widget.NewRadioGroup([]string{"Light", "Dark"}, func(value string) {
if value == "Light" {
app.Settings().SetTheme(theme.LightTheme())
} else {
app.Settings().SetTheme(theme.DarkTheme())
}
})
themeStyle.SetSelected("Light")
w.SetContent(container.NewVBox(
widget.NewLabel("Weight (kg):"),
weight,
widget.NewLabel("Height (m):"),
height,
widget.NewButton("Calculate", func() {
weightValue, err1 := strconv.ParseFloat(weight.Text, 64)
if err1 != nil {
fmt.Println("Error converting weight:", err1)
bmiText.SetText("Invalid weight input")
fmt.Println(err1)
return
}
heightValue, err := strconv.ParseFloat(height.Text, 64)
if err != nil {
fmt.Println("Error converting height:", err)
bmiText.SetText("Invalid height input")
fmt.Println(err)
return
}
bmi, category, tip := calculateBMI(weightValue, heightValue)
bmiText.SetText(fmt.Sprintf("Your BMI is: %.2f", bmi))
categoryText.SetText("Category: " + category)
tipText.SetText("Tip: " + tip)
// Update the UI
fmt.Println("Done!")
}),
bmiText,
categoryText,
tipText,
themeStyle,
))
w.ShowAndRun()
}
Explanation
- Imports: We import necessary packages including Fyne for the GUI components,
strconv
for converting string inputs to float, andfmt
for printing to the console. - calculateBMI Function: This function takes in the weight and height, calculates the BMI, and determines the health category and a tip based on the BMI. The function returns the BMI value, the category, and the tip.
- Main Function:
- We create a new Fyne app and window.
- The window size is set to 700×500 pixels.
- We define the input fields for weight and height, as well as labels to display the BMI, category, and tip.
- We also add a theme toggle that allows users to switch between light and dark modes.
- The “Calculate” button triggers the calculation when clicked, updating the labels with the results.
- Finally, we use
w.ShowAndRun()
to start the application.
Step 4: Running Your Application
Once you’ve written the code, it’s time to run your application.
- Run the Application:
- In your terminal, make sure you’re in your project directory and simply type:
go run main.go
- This will compile and run your BMI calculator.
- Interact with the Application:
- Enter your weight in kilograms and height in meters, then click “Calculate”.
- The application will display your BMI, the health category, and a helpful tip.
- You can also switch between light and dark themes using the radio buttons.
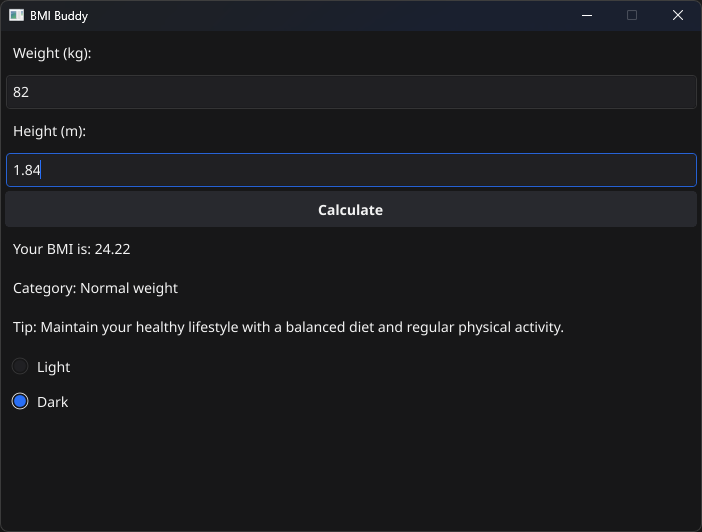
Conclusion
Congratulations! You’ve successfully created a simple BMI calculator with a GUI in Go using the Fyne library. Through this tutorial, you’ve learned the basics of setting up a Go environment, installing and using the Fyne library, and creating a functional desktop application with user interaction.
While this project is simple, it introduces you to the fundamentals of Go programming and GUI development, providing a foundation for more complex projects in the future. You can expand on this project by adding more features, such as saving user data, allowing for more input options, or even extending it to track BMI over time.
Go and Fyne together offer a powerful platform for building cross-platform desktop applications with ease. Keep experimenting and exploring the possibilities!
Happy coding!
References
- Official Go Programming Documentation
- Fyne GUI Documentation
- BMI Basics by CDC
- How to Install Go on Different Platforms
Go Programming, Fyne GUI, BMI Calculator, Go GUI Development, Golang Applications