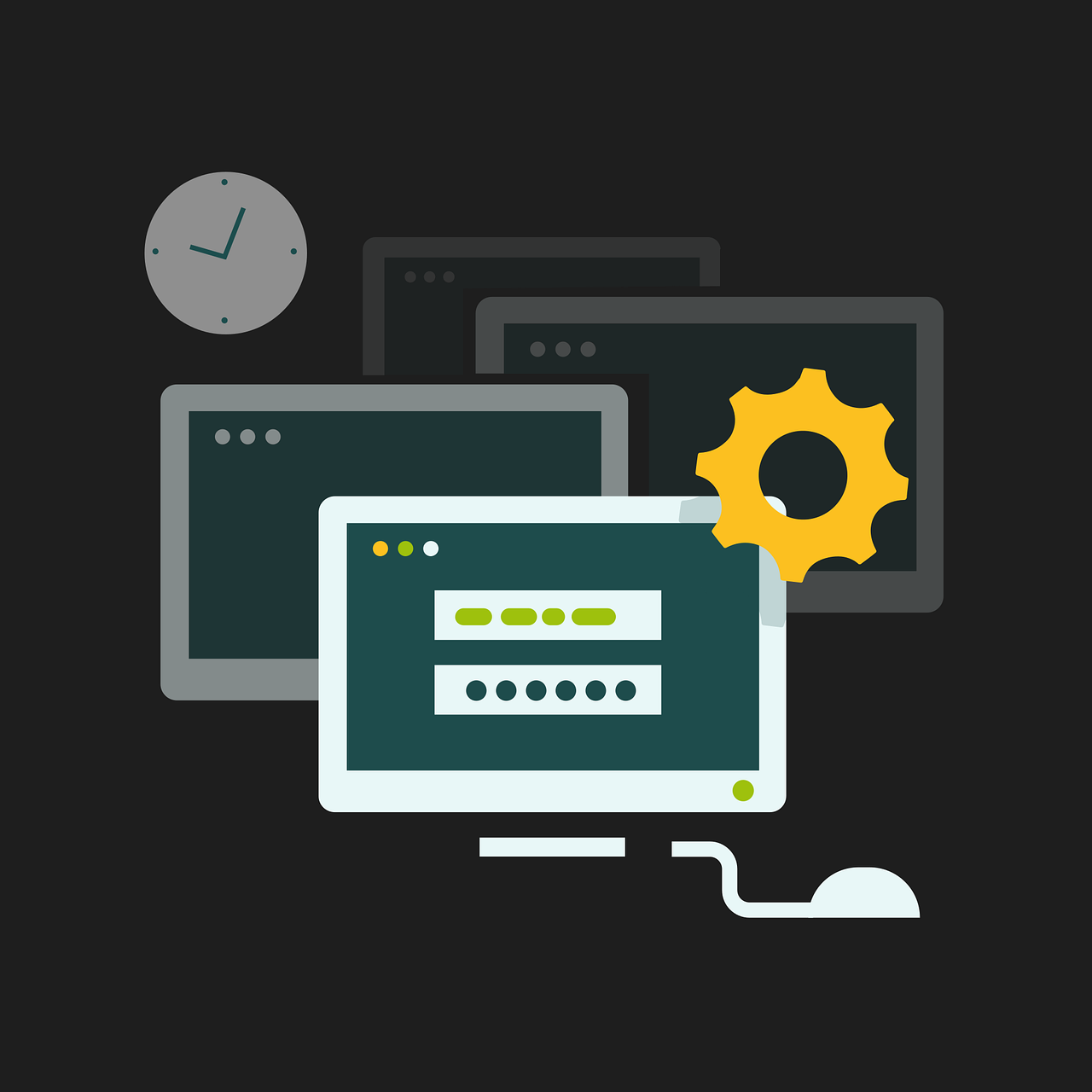
Introduction
Back-end development frameworks play a crucial role in creating robust, scalable, and efficient web applications, APIs, and services. These frameworks provide the structure and tools necessary to manage databases, handle requests, and perform business logic, allowing developers to focus on building functionality rather than reinventing the wheel. This article provides a detailed overview of back-end frameworks across multiple programming languages, highlighting their key features and providing official links for further exploration.
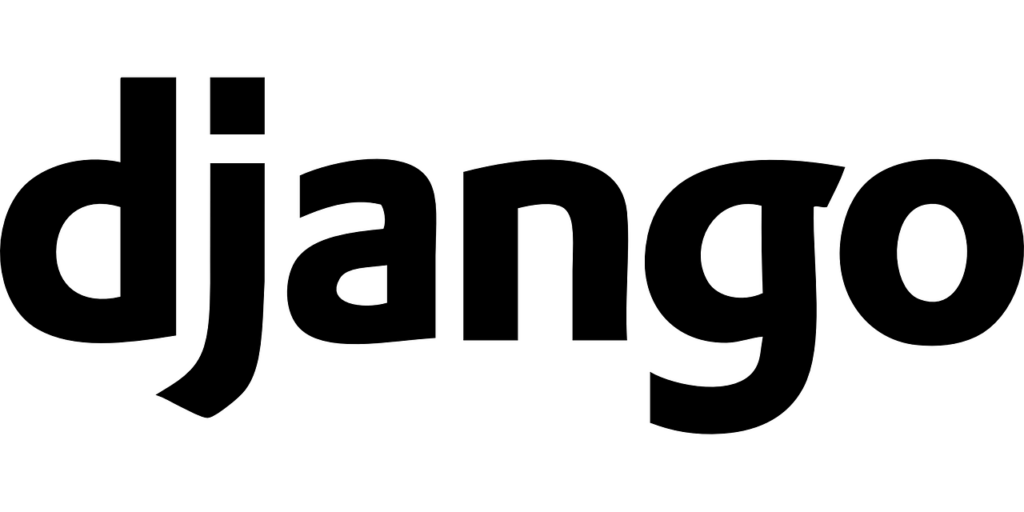
Detailed List of Back-End Frameworks by Programming Language
1. Python
Python is known for its readability and simplicity, making it a popular choice for back-end development. Here are some of the most widely-used Python back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Django | Full-stack, includes ORM, admin interface, built-in security features | Django |
Flask | Microframework, lightweight, flexible, supports extensions | Flask |
Pyramid | Flexible, modular, supports single-file apps to large projects | Pyramid |
FastAPI | Fast, high-performance, based on standard Python type hints, asynchronous support | FastAPI |
Tornado | Asynchronous networking library and web framework, supports long polling, WebSockets | Tornado |
Bottle | Very lightweight, single-file applications, no dependencies | Bottle |
Falcon | Minimalist, designed for building high-performance APIs, WSGI compliant | Falcon |
CherryPy | Object-oriented, minimalistic, runs on any operating system with Python installed | CherryPy |
Sanic | Asynchronous framework, built on Python 3.6+ features, very fast | Sanic |
Hug | API framework, designed to be as simple as possible, supports versioned APIs | Hug |
2. Java
Java is a powerful language with a strong presence in enterprise environments. Below are some popular Java back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Spring Boot | Convention over configuration, microservices ready, powerful ecosystem | Spring Boot |
Java EE | Standardized framework, enterprise features, JPA, EJB, CDI | Java EE |
Micronaut | JVM-based, microservices, reactive, cloud-native | Micronaut |
Dropwizard | Opinionated, includes Jetty, Jackson, Metrics, Hibernate, fast setup | Dropwizard |
Spark Java | Lightweight, expressive, minimalistic, ideal for microservices and small web apps | Spark Java |
JHipster | Full-stack generator, microservices, integrates with Angular/React | JHipster |
Quarkus | Kubernetes-native Java framework, tailored for GraalVM and OpenJDK HotSpot | Quarkus |
Play Framework | Reactive, full-stack, asynchronous, MVC architecture | Play Framework |
Vert.x | Polyglot, event-driven, reactive microservices, very scalable | Vert.x |
Helidon | Lightweight, cloud-native Java framework, supports reactive and imperative programming | Helidon |
3. JavaScript/Node.js
JavaScript, particularly with Node.js, has become a dominant force in back-end development due to its non-blocking, event-driven architecture. Below are popular Node.js frameworks:
Framework | Key Features | Official Link |
---|---|---|
Express.js | Minimalist, unopinionated, flexible, most popular Node.js framework | Express.js |
Koa.js | Lightweight, designed by the team behind Express.js, modern and modular | Koa.js |
NestJS | Progressive, uses TypeScript, modular, supports microservices | NestJS |
Sails.js | MVC framework, data-driven APIs, supports WebSockets, real-time applications | Sails.js |
Hapi.js | Rich plugin system, configuration-driven, powerful routing | Hapi.js |
AdonisJS | Full-stack, inspired by Laravel, includes ORM, authentication, and more | AdonisJS |
Meteor | Full-stack, real-time, integrated with MongoDB, supports React, Angular, and Vue | Meteor |
LoopBack | Highly extensible, focuses on APIs, built on top of Express.js | LoopBack |
Feathers.js | Microservices, real-time APIs, built on Express.js, supports REST and WebSockets | Feathers.js |
Strapi | Headless CMS, built with Node.js, API-first | Strapi |
4. Ruby
Ruby is known for its elegant syntax, and Ruby on Rails has become one of the most popular back-end frameworks in the world. Here are some Ruby back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Ruby on Rails | Convention over configuration, full-stack, includes ORM (ActiveRecord) | Ruby on Rails |
Sinatra | Minimalistic, DSL for creating simple web applications, microservices | Sinatra |
Hanami | Modular, lightweight, thread-safe, modern approach to MVC | Hanami |
Grape | REST-like API framework, lightweight, designed to run alongside other Rack applications | Grape |
Padrino | Built on top of Sinatra, full-featured, supports modular architecture | Padrino |
Cuba | Microframework, inspired by Sinatra, very lightweight | Cuba |
Ramaze | Simple, flexible, fast, includes templating and session management | Ramaze |
Camping | Minimalistic, fits in a single file, inspired by Sinatra | Camping |
Roda | Lightweight, scalable, designed to build web applications that are fast and secure | Roda |
Rails API | Lightweight, Rails-based, focused on building APIs | Rails API |
5. PHP
PHP remains one of the most widely-used languages for web development, particularly for server-side scripting. Below are some popular PHP back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Laravel | Elegant syntax, MVC architecture, extensive ecosystem, built-in ORM | Laravel |
Symfony | Reusable PHP components, modular, enterprise-level | Symfony |
CodeIgniter | Lightweight, simple to use, small footprint | CodeIgniter |
Yii Framework | High-performance, component-based PHP framework | Yii Framework |
Phalcon | High-performance, low-level, written in C, very fast | Phalcon |
Zend Framework | Enterprise-grade, object-oriented, comprehensive set of features | Zend Framework |
CakePHP | Rapid development framework, scaffolding, easy to use | CakePHP |
Slim Framework | Micro framework, simple yet powerful, very flexible | Slim Framework |
Laminas | Enterprise-level, continuation of Zend Framework, modular | Laminas |
FuelPHP | Full-featured PHP framework, modular, security-focused | FuelPHP |
6. C#
C# is predominantly used within the .NET ecosystem for building web applications, APIs, and services. Below are some popular C# back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
ASP.NET Core | Cross-platform, high-performance, built on .NET, supports REST APIs, MVC, and Razor Pages | ASP.NET Core |
Nancy | Lightweight, inspired by Sinatra, highly modular, supports OWIN | Nancy |
ServiceStack | Simple, fast, API-first, built-in support for REST and JSON | ServiceStack |
Web API | Framework for building HTTP services, part of the ASP.NET framework | Web API |
WCF | Framework for building service-oriented applications, supports various protocols and transports | WCF |
Blazor Server | Allows building interactive web UIs with C#, supports WebAssembly | Blazor Server |
DotVVM | MVVM framework for web apps, based on ASP.NET Core, supports WebAssembly | DotVVM |
Orchard Core | Modular, multi-tenant CMS framework, built on ASP.NET Core | Orchard Core |
Umbraco | Open-source CMS, built on ASP.NET, very flexible and extensible | Umbraco |
OpenRasta | Framework for building web-based APIs, extensible, supports content negotiation | OpenRasta |
7. JavaScript/TypeScript (Node.js)
JavaScript, with its Node.js runtime, is extremely popular for back-end development, especially for building scalable network applications. TypeScript is a typed superset of JavaScript, providing additional features. Below are popular frameworks for Node.js/TypeScript:
Framework | Key Features | Official Link |
---|---|---|
Express.js | Minimalist, unopinionated, flexible, most popular Node.js framework | Express.js |
NestJS | Progressive, uses TypeScript, modular, supports microservices | NestJS |
Koa.js | Lightweight, designed by the team behind Express.js, modern and modular | Koa.js |
Sails.js | MVC framework, data-driven APIs, supports WebSockets, real-time applications | Sails.js |
LoopBack | Highly extensible, focuses on APIs, built on top of Express.js | LoopBack |
Meteor | Full-stack, real-time, integrated with MongoDB, supports React, Angular, and Vue | Meteor |
Hapi.js | Rich plugin system, configuration-driven, powerful routing | Hapi.js |
AdonisJS | Full-stack, inspired by Laravel, includes ORM, authentication, and more | AdonisJS |
Feathers.js | Microservices, real-time APIs, built on Express.js, supports REST and WebSockets | Feathers.js |
Strapi | Headless CMS, built with Node.js, API-first | Strapi |
8. Go
Go, or Golang, is known for its simplicity, performance, and concurrency model, making it a popular choice for building back-end services and APIs. Here are some popular Go frameworks:
Framework | Key Features | Official Link |
---|---|---|
Gin | Lightweight, high-performance, supports middleware and routing | Gin |
Echo | Minimalistic, high-performance, includes built-in support for JWT, CORS, and more | Echo |
Beego | Full-featured, built-in ORM, web framework and application development toolkit | Beego |
Revel | Full-featured, batteries-included web framework, inspired by Rails | Revel |
Fiber | Inspired by Express.js, fast, minimalistic, built on Fasthttp | Fiber |
Buffalo | Full-featured, hot-reloading, includes web framework and tools for development | Buffalo |
Chi | Lightweight, idiomatic, built with a focus on composability and modularity | Chi |
Iris | Full-featured, built-in MVC support, rich routing, highly performant | Iris |
Martini | Lightweight, simple to use, but with enough power for most web applications | Martini |
Gorilla | Modular, provides tools like mux, sessions, web sockets, and more | Gorilla |
9. Ruby
Ruby is known for its elegant syntax, and Ruby on Rails has become one of the most popular back-end frameworks in the world. Here are some Ruby back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Ruby on Rails | Convention over configuration, full-stack, includes ORM (ActiveRecord) | Ruby on Rails |
Sinatra | Minimalistic, DSL for creating simple web applications, microservices | Sinatra |
Hanami | Modular, lightweight, thread-safe, modern approach to MVC | Hanami |
Grape | REST-like API framework, lightweight, designed to run alongside other Rack applications | Grape |
Padrino | Built on top of Sinatra, full-featured, supports modular architecture | Padrino |
Cuba | Microframework, inspired by Sinatra, very lightweight | Cuba |
Ramaze | Simple, flexible, fast, includes templating and session management | Ramaze |
Camping | Minimalistic, fits in a single file, inspired by Sinatra | Camping |
Roda | Lightweight, scalable, designed to build web applications that are fast and secure | Roda |
Rails API | Lightweight, Rails-based, focused on building APIs | Rails API |
10. Rust
Rust is known for its memory safety and performance, making it an excellent choice for systems programming, including back-end services and APIs. Below are some popular Rust frameworks:
Framework | Key Features | Official Link |
---|---|---|
Rocket | Full-featured, type-safe, built-in features for request handling, databases, and more | Rocket |
Actix Web | Powerful, pragmatic, actor-based framework, supports async programming | Actix Web |
Warp | Simple, composable, built on top of tokio, supports WebSockets, file serving, etc. | Warp |
Tide | Minimalistic, built on async-std, modular middleware, flexible | Tide |
Gotham | Flexible, built for async, middleware-centric | Gotham |
Nickel | Inspired by Express.js, simple and easy to use, middleware-based | Nickel |
Axum | Web framework that focuses on ergonomics and productivity, built on hyper and tower | Axum |
Iron | Extensible, focus on middleware, inspired by Sinatra | Iron |
Salvo | Web server framework with expressive routing, middleware support | Salvo |
Thruster | Fast, built with async I/O, middleware-based | Thruster |
11. PHP
PHP remains one of the most widely-used languages for web development, particularly for server-side scripting. Below are some popular PHP back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Laravel | Elegant syntax, MVC architecture, extensive ecosystem, built-in ORM | Laravel |
Symfony | Reusable PHP components, modular, enterprise-level | Symfony |
CodeIgniter | Lightweight, simple to use, small footprint | CodeIgniter |
Yii Framework | High-performance, component-based PHP framework | Yii Framework |
Phalcon | High-performance, low-level, written in C, very fast | Phalcon |
Zend Framework | Enterprise-grade, object-oriented, comprehensive set of features | Zend Framework |
CakePHP | Rapid development framework, scaffolding, easy to use | CakePHP |
Slim Framework | Micro framework, simple yet powerful, very flexible | Slim Framework |
Laminas | Enterprise-level, continuation of Zend Framework, modular | Laminas |
FuelPHP | Full-featured PHP framework, modular, security-focused | FuelPHP |
12. Swift
Swift is Apple’s language for developing iOS and macOS applications, but it’s also used in server-side development with frameworks like Vapor. Below are some popular Swift back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Vapor | Lightweight, modular, built on Swift, designed for HTTP/2, WebSockets, and more | Vapor |
Kitura | IBM-backed, cross-platform, supports multiple protocols, includes middleware | Kitura |
Perfect | Server-side Swift framework, includes built-in support for routing, ORM, and templating | Perfect |
Zewo | Modular, HTTP server and client, WebSocket support, written in Swift | Zewo |
SwiftNIO | Low-level networking framework, built for performance, used to build custom servers | SwiftNIO |
Hummingbird | Lightweight, minimalistic, powered by SwiftNIO | Hummingbird |
Smoke Framework | Server-side framework, designed by Amazon for Swift, includes AWS integrations | Smoke Framework |
Tiberius | Simple, fast, and flexible microframework for Swift | Tiberius |
Ambassador | Swift middleware framework, inspired by Rack and Express.js | Ambassador |
Expressible | Express.js-like microframework for Swift, easy to use and minimal | Expressible |
13. Elixir
Elixir is known for its concurrency and scalability, particularly with the Phoenix framework. Below are some popular Elixir back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Phoenix | Built on Elixir, focuses on performance and productivity, includes channels for real-time features | Phoenix |
Plug | Specification and composable modules for web applications, similar to Rack for Ruby | Plug |
Nerves | Framework for creating embedded systems, built on Elixir, supports IoT development | Nerves |
Sugar | Syntactic sugar on top of Plug, focuses on productivity | Sugar |
Maru | Elixir-based API microframework, built on Plug | Maru |
Absinthe | GraphQL toolkit for Elixir, fully featured, works with Phoenix | Absinthe |
Trot | Micro framework for building REST APIs, built on Plug | Trot |
Raxx | Web interface for Elixir, focuses on clarity and simplicity | Raxx |
Drab | Phoenix framework extension, allows you to run Elixir code on the client side | Drab |
Bandit | Pure Elixir web server, built with modern concurrency in mind | Bandit |
14. Clojure
Clojure is a modern Lisp dialect that runs on the JVM, and it’s known for its functional programming features. Here are some popular Clojure back-end frameworks:
Framework | Key Features | Official Link |
---|---|---|
Ring | Minimalist, acts as the foundation for other frameworks, inspired by Rack for Ruby | Ring |
Compojure | Routing library for Ring, easy-to-use DSL, composes well with other libraries | Compojure |
Luminus | Full-featured, built on top of Ring and Compojure, focuses on simplicity and ease of use | Luminus |
Pedestal | Highly modular, built on Ring, focuses on real-time and asynchronous processing | Pedestal |
Liberator | Library for building RESTful APIs, built on Ring, focuses on compliance with REST principles | Liberator |
Duct | Minimalistic, modular, built on top of Ring and Integrant, used for creating maintainable applications | Duct |
Reitit | Fast data-driven routing library for Ring, pluggable, modular | Reitit |
Hoplon | Web framework and templating engine, supports client-server communication | Hoplon |
Fulcro | Full-stack framework, supports REST and GraphQL, built on top of Om and React | Fulcro |
Bidi | Bidirectional routing, works with Ring, can map routes to URLs and back | Bidi |
15. Perl
Perl, known for its strengths in text processing and scripting, also has back-end frameworks for building web applications:
Framework | Key Features | Official Link |
---|---|---|
Mojolicious | Real-time web framework, non-blocking, event-driven | Mojolicious |
Dancer | Lightweight web application framework, simple DSL | Dancer |
Catalyst | MVC web framework, highly flexible and modular | Catalyst |
Perl CGI | Standard library for web applications, simple and straightforward | CGI.pm |
Mason | Web application framework, integrates with Apache | Mason |
Plack | Toolkit for building web applications, middleware, PSGI-based | Plack |
Jifty | Web application framework, focuses on rapid development | Jifty |
Squatting | Minimalistic web framework, built on PSGI, uses HTTP::Engine | Squatting |
Maypole | MVC web application framework, integrates with Class::DBI and Template Toolkit | Maypole |
Amon2 | Lightweight, pluggable web application framework, inspired by Sinatra | Amon2 |
Conclusion
This table provides a comprehensive overview of 80 back-end frameworks across multiple programming languages, offering developers a wide range of options for building robust and scalable web applications, APIs, and services. Whether you’re working with Python, Java, JavaScript, Ruby, or any other language listed here, this guide should help you find the right framework for your project.
By exploring the official links provided, you can delve deeper into each framework’s features and capabilities, ensuring you choose the best option for your next back-end development project.
Reference Section
For further reading on back-end development frameworks, refer to these external links:
- Django Official Documentation
- Express.js Official Documentation
- Spring Boot Official Guide
- Phoenix Framework
- Rocket Framework (Rust)
- Understanding Go Concurrency
Tags: Back-end development, programming languages, web frameworks, server-side frameworks, API development, open-source frameworks
No comment yet, add your voice below!