“Managing large collections of audio files can be a daunting task, especially when dealing with thousands of files spread across multiple folders and subdirectories. Traditional tools often fall short when it comes to preserving directory structures or efficiently processing bulk conversions. Bison Audio Converter addresses these challenges by offering an intuitive solution that automates the entire process. With its powerful batch conversion capabilities, you can convert entire directories while maintaining the original folder hierarchy. This is particularly useful for professionals in the music, podcasting, or archiving industries, where preserving the organization of files is critical.
This article explores the features of Bison Audio Converter, the code structure, and demonstrates how you can build and use it.
π Features
- Batch Conversion: Batch conversion allows you to process entire directories of audio files in one go. Whether you have a folder with a few dozen files or a complex structure with tens of thousands, Bison Audio Converter handles the workload effortlessly. This feature is invaluable for scenarios like converting a music library, archiving old recordings, or preparing audio assets for a project. The tool maintains the original folder structure, so you donβt have to spend time reorganizing files after conversion.
- Single File Conversion: Sometimes, you only need to convert a single file, and Bison Audio Converter provides a streamlined process for that. This is perfect for quick tasks like converting a new recording or testing the quality of a specific audio format. The single-file conversion interface is simple and efficient, saving you from the hassle of configuring settings for batch jobs.
- Supported Formats: Bison Audio Converter supports WAV as the input format and both WAV and MP3 as output formats. These formats cover a wide range of use cases, from high-quality archival storage (WAV) to compressed formats suitable for web distribution or portable devices (MP3). The extensible design means additional formats can be integrated with minimal changes to the codebase.
– Input: WAV
– Output: MP3, WAV
- ImGui-based GUI: A responsive and lightweight interface built with ImGui.
- Real-time Logging: See real-time progress and logs during conversion.
- Cross-Platform: Compatible with both Windows and Linux.
- Customizable Layouts: Default window positions and flexible layouts for ease of use.
π οΈ Building the Project
Prerequisites
Ensure you have the following tools installed:
- CMake (version 3.16 or higher)
- LLVM/Clang or your preferred C++ compiler
- vcpkg for dependency management
Install the necessary libraries via vcpkg
:
vcpkg install imgui["sfml-binding"] fmt sfml libvorbis mp3lame miniaudio
Building Steps
- Clone the Repository:
git clone https://github.com/rambod/BisonAudioConverter.git
cd BisonAudioConverter
- Set Up the Build Directory:
cmake -S . -B cmake-build-debug -DCMAKE_TOOLCHAIN_FILE=C:/vcpkg/scripts/buildsystems/vcpkg.cmake
- Build the Project:
cmake --build cmake-build-debug
- Run the Executable:
./cmake-build-debug/BisonAudioConverter
π₯οΈ User Interface Overview
Main Sections
The interface is designed with simplicity in mind. When you launch Bison Audio Converter, you’ll see three main sections:
- Bulk Audio Converter
- Input Directory: Click the ‘Select’ button to choose the folder containing your audio files.
- Output Directory: Choose where the converted files should be saved.
- Output Format: Select either ‘WAV’ or ‘MP3’.
- Start: Click ‘Start Conversion’ to begin processing.
- Single File Converter
- Similar to the bulk converter but designed for individual files.
- Log Window
- Displays detailed progress and error messages, keeping you informed.
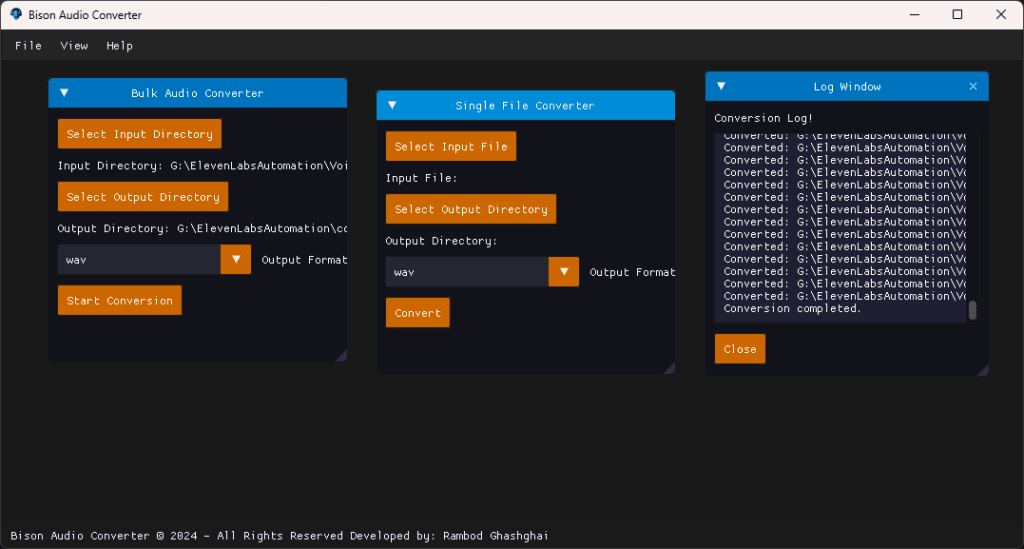
π How to Use Bison Audio Converter
π Bulk Audio Conversion
The bulk conversion feature allows you to process entire folders of audio files while maintaining the original directory structure.
- Select Input Directory:
- Click the “Browse” button next to the Input Directory field.
- Navigate to the folder containing your audio files and select it.
- Bison Audio Converter will process all supported files (currently
.wav
) within this folder and its subdirectories.
- Select Output Directory:
- Click the “Browse” button next to the Output Directory field.
- Choose the destination folder where you want the converted files to be saved.
- The original folder structure will be mirrored in the output directory.
- Choose Output Format:
- Select the desired format for the output files (
WAV
orMP3
). - This option determines how the files will be encoded during conversion.
- Select the desired format for the output files (
- Start Conversion:
- Click the “Start Conversion” button to begin processing.
- The tool will display the progress and any errors in the Log Window.
- You can monitor which files are being processed and the status of each conversion.
- Completion:
- Once all files are processed, a completion message will appear in the log.
- Review the output folder to verify the converted files.
π΅ Single File Conversion
The single file conversion feature is ideal for quick, one-off tasks when you only need to process a single audio file.
- Select Input File:
- Click the “Browse” button next to the Input File field.
- Choose the specific
.wav
file you want to convert.
- Select Output Directory:
- Click the “Browse” button next to the Output Directory field.
- Specify the folder where the converted file should be saved.
- Choose Output Format:
- Select the desired format for the output file (
WAV
orMP3
).
- Select the desired format for the output file (
- Convert File:
- Click the “Convert” button to start the conversion.
- The progress will be displayed in the Log Window.
- A success message will appear once the file is converted.
- Check Output:
- Open the output folder to find the converted file.
π Log Window
The Log Window is a critical part of the interface for monitoring and debugging.
- Real-time Feedback:
- As each file is processed, the log will display status messages (e.g., “Converting file.wav to file.mp3”, “Conversion completed”, “Error: File not found”).
- This helps you track progress and identify any issues.
- Customizable Position:
- You can reposition the log window within the interface to suit your workflow.
- Error Reporting:
- Any errors encountered during conversion are displayed in red for easy identification.
By using the Log Window, you stay informed about the entire conversion process and can quickly address any problems that arise.
π Code Breakdown
1. Main Logic: AudioConverter.cpp
The core functionality for audio conversion resides here. Letβs examine some key functions:
Finding Audio Files
std::vector<std::string> AudioConverter::FindAudioFiles(const std::string& directory) {
const std::vector<std::string> SUPPORTED_EXTENSIONS = {".wav"};
std::vector<std::string> audioFiles;
for (const auto& entry : fs::recursive_directory_iterator(directory)) {
if (entry.is_regular_file() &&
std::find(SUPPORTED_EXTENSIONS.begin(), SUPPORTED_EXTENSIONS.end(), entry.path().extension().string()) != SUPPORTED_EXTENSIONS.end()) {
audioFiles.push_back(entry.path().string());
}
}
return audioFiles;
}
Converting to MP3
bool AudioConverter::ConvertToMP3(const std::string& inputFile, const std::string& outputFile) {
// Conversion code using miniaudio and LAME libraries
}
2. Bulk Conversion UI: MainWindow.cpp
Handles the user interface for bulk conversion.
π Get Bison Audio Converter
π₯ Download the Latest Release
You can download the latest version of Bison Audio Converter directly:
β‘οΈ Download Bison Audio Converter (Setup.exe)
ποΈ Explore the Project on GitHub
Check out the source code, report issues, and contribute to the project:
β‘οΈ Bison Audio Converter on GitHub
π More Projects and Insights
Visit my personal website for more projects, insights, and tutorials:
π rambod.net
Happy converting! π