Raylib Tutorial Introduction
Raylib tutorial: Raylib is a simple and easy-to-use library for game development and multimedia programming in C. It’s perfect for beginners and those who want to create 2D and 3D games without getting bogged down in complex setups. In this guide, we’ll walk you through installing Raylib using vcpkg and setting it up in CLion. We’ll also create a simple project that draws and moves a rectangle around the screen. The focus is on keeping the setup and code as easy and straightforward as possible.
Prerequisites
Before you begin, ensure you have the following installed:
- CLion (or any other IDE that supports CMake)
- CMake (usually bundled with CLion)
- vcpkg (C++ package manager)
Step 1: Install vcpkg
If you haven’t installed vcpkg yet, follow these steps:
- Clone the vcpkg repository:
git clone https://github.com/microsoft/vcpkg.git
cd vcpkg
- Bootstrap vcpkg: On Windows:
.\bootstrap-vcpkg.bat
On macOS/Linux:
./bootstrap-vcpkg.sh
- Integrate vcpkg with your IDE:
./vcpkg integrate install
This integration allows vcpkg to work seamlessly with CMake in your IDE.
Step 2: Install Raylib with vcpkg
With vcpkg installed, you can easily install Raylib:
vcpkg install raylib
This command downloads and builds Raylib and its dependencies, making them available for use in your projects.
Step 3: Create a New C++ Project in CLion
- Open CLion and create a new C++ project.
- Choose “CMake” as your project type.
- Set your project name and directory.
Once the project is created, you’ll see a CMakeLists.txt
file. We’ll modify this file in the next step.
Step 4: Configure CMakeLists.txt
To use Raylib in your project, you need to link it properly using CMake. Here’s how to configure your CMakeLists.txt
file:
cmake_minimum_required(VERSION 3.10)
project(RaylibExample)
# Set C++ standard
set(CMAKE_CXX_STANDARD 17)
# Add the raylib package via vcpkg
find_package(raylib CONFIG REQUIRED)
# Add the executable
add_executable(RaylibExample main.cpp)
# Link Raylib
target_link_libraries(RaylibExample PRIVATE raylib::raylib)
This configuration does the following:
- Sets the C++ standard to C++17.
- Finds the Raylib package installed by vcpkg.
- Links the Raylib library to your project.
Step 5: Write the Code
Now, let’s create a simple C++ program that initializes a window and draws a rectangle that you can move around with the arrow keys. Create a main.cpp
file with the following content:
#include <raylib.h>
int main() {
// Initialize the window
InitWindow(800, 600, "Raylib Example");
// Define the rectangle
Rectangle rect = { 350, 250, 100, 100 };
int speed = 5;
// Main game loop
while (!WindowShouldClose()) {
// Move the rectangle with arrow keys
if (IsKeyDown(KEY_RIGHT)) rect.x += speed;
if (IsKeyDown(KEY_LEFT)) rect.x -= speed;
if (IsKeyDown(KEY_DOWN)) rect.y += speed;
if (IsKeyDown(KEY_UP)) rect.y -= speed;
// Start drawing
BeginDrawing();
ClearBackground(RAYWHITE);
// Draw the rectangle
DrawRectangleRec(rect, BLUE);
DrawText("Move the rectangle with arrow keys", 10, 10, 20, DARKGRAY);
// End drawing
EndDrawing();
}
// Close the window
CloseWindow();
return 0;
}
Step 6: Build and Run the Project
- Build the Project: In CLion, click on the build button or press
Ctrl + F9
to build your project. - Run the Project: After building, run the project with
Shift + F10
or by clicking the run button.
When you run the program, you should see a window open with a rectangle in the center. You can move the rectangle around using the arrow keys.
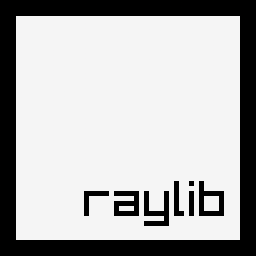
Conclusion
By following these steps, you’ve set up a simple C++ project using Raylib in CLion with minimal effort. With vcpkg handling the dependencies and CMake managing the build process, you can focus on coding your game or multimedia project without worrying about complex configurations.
Raylib is an excellent choice for beginners or anyone looking to quickly prototype and develop 2D or 3D applications in C++. The combination of CLion, vcpkg, and Raylib offers a streamlined workflow that makes development smooth and enjoyable.
Now that you’ve got the basics down, you can start exploring more features of Raylib and begin building your own games and interactive applications!
References
- Raylib Official Website
Raylib Official Documentation
Explore the official documentation for Raylib to understand its features, installation process, and various examples. - vcpkg Official Repository
vcpkg on GitHub
Check out the vcpkg repository on GitHub for detailed instructions on how to install and use this C++ package manager. - CLion IDE
CLion Official Website
Visit the official website of CLion to learn more about this powerful C++ IDE and its features. - Raylib GitHub Repository
Raylib on GitHub
Explore the source code of Raylib on GitHub, contribute to the project, or review various examples created by the community. - Guide to 100 Game Development Frameworks, Libraries, and Engines Across Popular Programming Languages